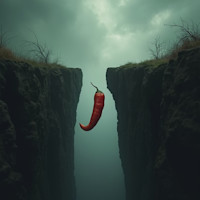
Jackson and Lombok @Builder pitfall
Lombok has become a default library on any of my projects. However, any new power comes with great responsibility and understanding its inner working is a necessity. In this blogpost we will cover one pitfall that will likely arise if you use Jackson and the @Builder pattern.
# Introduction
Besides being an island in Indonesia
Lombok
is an elegant java project (hence the name) meant to reduce boilerplate code and make any Java developers life and productivity a bit better.
<dependencies>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
</dependencies>
Lombok
is an annotation processor and therefore effectively generating boilerplate code at build time. I won't argue against the fact that it can be scary for some, especially around security aspects. However the project is managed by serious people having clear
security policies
.
I've now been using
Lombok
in production for more than a decade and I would recommend it a hundred times to anyone still reluctant to it.
# Jackson & Lombok
A frequent use case is to use Lombok on the API domain model which may rely on Jackson for any JSON (de)serilisation.
Considering making your domain model or any of your model immutable is most probably one of the most important and beneficial move to make. Not only will it make your code cleaner but it will surely save you hundred of hours of debugging on the long term at the cost of a small in-memory footprint overhead.
As a study case, let's consider an API that consumes and saves a
Person
.
@RestController
public class PersonController {
@PostMapping("/persons")
@ResponseStatus(HttpStatus.CREATED)
public Person save(@RequestBody Person person) {
// delegate creation
System.out.printf("Saved person [%s]%n", person);
return person;
}
@Getter
@Builder
@ToString
public static class Person {
private final Gender gender;
private final String name;
private final LocalDate birthDate;
enum Gender {
M,
F,
U
}
}
}
A
Person
is guaranteed to be immutable as it doesn't define any
@Setter
but only a single
@Builder
.
Saving a
Person
would simply require a call such as this one.
curl --location 'http://localhost:8080/persons' \
--header 'Content-Type: application/json' \
--data '{
"gender": "M",
"name": "John Doe",
"birthDate": "1953-02-25"
}'
Saved person [PersonController.Person(gender=M, name=John Doe, birthDate=1953-02-25)]
And this, actually works. However, if you are a Lombok connoisseur you probably noticed that something is amiss. In order to pinpoint what's wrong, let's improve our Person class by adding a
@Default
behaviour when the gender is missing.
@Getter
@Builder
@ToString
public static class Person {
@Builder.Default
private final Gender gender = Gender.U;
private final String name;
private final LocalDate birthDate;
enum Gender {
M,
F,
U
}
}
In this situation, if
gender
is missing in the payload the object should be built with
Gender.U
as a fallback value.
curl --location 'http://localhost:8080/persons' \
--header 'Content-Type: application/json' \
--data '{
"name": "John Doe",
"birthDate": "1953-02-25"
}'
Saved person [PersonController.Person(gender=null, name=John Doe, birthDate=1953-02-25)]
So what went wrong here?
Jackson
is smart and perhaps sometimes a bit too clever. The question to ask is how does Jackson know it must use the
@Builder
for deserialisation? If you look at Jackson
documentation
you need to instruct the binder to rely on the builder by adding
@JsonDeserialize(builder = ...)
annotation.
So what is happening here? How is Jackson able to deserialise the object at all? We didn't declare any
@Setter
, and there is obviously no default constructors.
Delombokify
the
@Builder
annotation we will help to understand what is going on.
@Getter
@Builder
@ToString
public static class Person {
@Builder.Default
private final Gender gender = Gender.U;
private final String name;
private final LocalDate birthDate;
Person(PersonController.Person.Gender gender, String name, LocalDate birthDate) {
this.gender = gender;
this.name = name;
this.birthDate = birthDate;
}
private static Gender $default$gender() {return Gender.U;}
public static PersonBuilder builder() {return new PersonBuilder();}
enum Gender {
M,
F,
U
}
public static class PersonBuilder {
private Gender gender$value;
private boolean gender$set;
private String name;
private LocalDate birthDate;
PersonBuilder() {}
public PersonBuilder gender(PersonController.Person.Gender gender) {
this.gender$value = gender;
this.gender$set = true;
return this;
}
public PersonBuilder name(String name) {
this.name = name;
return this;
}
public PersonBuilder birthDate(LocalDate birthDate) {
this.birthDate = birthDate;
return this;
}
public Person build() {
Gender gender$value = this.gender$value;
if (!this.gender$set) {
gender$value = Person.$default$gender();
}
return new Person(gender$value, this.name, this.birthDate);
}
public String toString() {
return "PersonController.Person.PersonBuilder(gender$value=" + this.gender$value + ", name=" + this.name + ", birthDate=" + this.birthDate
+ ")";
}
}
}
⚠️ In order to eventually build the object a
package
protected constructor
is generated. Despite not being
public
Jackson is clever enough to access it.
✅ Consequently to fix our domain model we need to introduce
@Jacksonized
annotation from Lombok which will in turn generate the missing
@JsonDeserialize
annotation and instruct properly Jackson for deserialisation.
@Getter
@Builder
@Jacksonized
@ToString
public static class Person {
@Builder.Default
private final Gender gender = Gender.U;
private final String name;
private final LocalDate birthDate;
enum Gender {
M,
F,
U
}
}
curl --location 'http://localhost:8080/persons' \
--header 'Content-Type: application/json' \
--data '{
"name": "John Doe",
"birthDate": "1953-02-25"
}'
Saved person [PersonController.Person(gender=U, name=John Doe, birthDate=1953-02-25)]
# Wrap up
Lombok helps you to reduce tons of boilerplate code in exchange of a few added annotations. However if not controlled or understood properly it may lead to unexpected behaviours.
If
@Builder
pattern is used on domain model objects in conjunction with Jackson as a deserialiser framework then
always
combine
@Builder
and
@Jacksonized
together.
The project can be found on my
github
.
Leave a thumbs up
Leave a thumbs up if you liked it