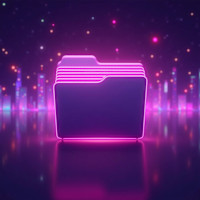
Quarkus and Maven multi-modules pitfalls
Building an application with Quarkus using Maven multi-modules can be tricky due to its native build features. In this article, we’ll explore some helpful strategies to avoid common pitfalls and ensure a smooth development experience.
# Maven Multi-modules pitfalls with Quarkus
Quarkus provides a variety of
guides
to help you get started, but it doesn’t offer a standardized, production-ready folder structure. Personally, I’ve grown accustomed to organizing my code with Maven in a mix of domain and layered structure. It helps me reducing my cognitive load and encourages better code decoupling. I won't start a DDD debate and whether this is good or bad, it is just what I find suitable.
# Code structure
Let's consider the following Maven module structure
<modules>
<module>component</module> <!-- main module (deployable) -->
<module>model</module> <!-- domain model layer -->
<module>service</module> <!-- service layer -->
<module>client</module> <!-- external communication (e.g. HTTP clients) -->
</modules>
# Pitfalls
When developing with Quarkus, you’ll quickly encounter different
runtime
modes:
- quarkus:dev for hot reload
- Uber-jar (JVM)
- Native (GraalVM)
While your application might run smoothly in development mode, that doesn’t necessarily guarantee the same seamless execution in native mode. The behavior can also vary between mono and multi-module setups (especially in dev mode).
# quarkus-maven-module
If
quarkus-maven-module
is defined on the parent pom.xml you may encounter errors at build time in
-Pnative
mode.
blog
├── model
│ ├── src
│ │ │── main
| └── pom.xml
| ...
└── pom.xml <!-- defines quarkus-maven-module plugin -->
quarkus-maven-module
is doing a whole lot of magic and if this plugin is executed on a module that is actually not a quarkus modules it will
complain on the docs generation
.
[1/7] Initializing...
Error: Feature io.quarkus.runtime.graal.DisableLoggingFeature class not found on the classpath. Ensure that the name is correct and that the class is on the classpath.
com.oracle.svm.core.util.UserError$UserException: Feature io.quarkus.runtime.graal.DisableLoggingFeature class not found on the classpath. Ensure that the name is correct and that the class is on the classpath.
at org.graalvm.nativeimage.builder/com.oracle.svm.core.util.UserError.abort(UserError.java:73)
at org.graalvm.nativeimage.builder/com.oracle.svm.hosted.FeatureHandler.registerFeatures(FeatureHandler.java:175)
at org.graalvm.nativeimage.builder/com.oracle.svm.hosted.NativeImageGenerator.setupNativeImage(NativeImageGenerator.java:852)
at org.graalvm.nativeimage.builder/com.oracle.svm.hosted.NativeImageGenerator.doRun(NativeImageGenerator.java:575)
at org.graalvm.nativeimage.builder/com.oracle.svm.hosted.NativeImageGenerator.run(NativeImageGenerator.java:535)
at org.graalvm.nativeimage.builder/com.oracle.svm.hosted.NativeImageGeneratorRunner.buildImage(NativeImageGeneratorRunner.java:403)
at org.graalvm.nativeimage.builder/com.oracle.svm.hosted.NativeImageGeneratorRunner.build(NativeImageGeneratorRunner.java:580)
at org.graalvm.nativeimage.builder/com.oracle.svm.hosted.NativeImageGeneratorRunner.main(NativeImageGeneratorRunner.java:128)
One way would be to pull
quarkus-maven-module
plugin from the parent to the concerned modules, another alternative would be to disable it where not required.
// parent > pom.xml
<properties>
...
<skip.quarkus.build>false</skip.quarkus.build>
...
</properties>
<plugin>
<groupId>io.quarkus.platform</groupId>
<artifactId>quarkus-maven-plugin</artifactId>
...
<configuration>
<skip>${skip.quarkus.build}</skip>
</configuration>
</plugin>
// parent > model > pom.xml
<properties>
<skip.quarkus.build>true</skip.quarkus.build>
</properties>
# CDI in quarkus:dev
When it comes to developer experience, Quarkus stands out with its unmatched features—instantaneous hot reload, lightning-fast startup times, and seamless integration with its web stack (Qute). No tool, whether it’s JRebel, Spring Boot DevTools, or even the classic Play! Framework, quite matches the smooth workflow Quarkus offers.
However, in a multi-module setup, things get more complicated. Bean discovery becomes trickier and needs a little nudge in order to work.
To simplify
bean discovery
without introducing significant overhead, a simple yet effective solution is to use the
Jandex Maven plugin
. This plugin automatically generates a bean index that Quarkus can rely on, helping to streamline the process. There might still be some small issues on dev mode that may require a fresh restart now on then, but I'm so far really pleased with the development experience.
<plugin>
<groupId>io.smallrye</groupId>
<artifactId>jandex-maven-plugin</artifactId>
<version>${jandex-plugin.version}</version>
<executions>
<execution>
<id>make-index</id>
<goals>
<goal>jandex</goal>
</goals>
</execution>
</executions>
</plugin>
# Curious to see how everything is wired up?
For a closer look at the setup on gdevxy.ch, check out the project on
GitHub
!
Leave a thumbs up
Leave a thumbs up if you liked it