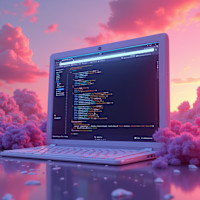
Playwright Integration Testing with Quarkus
Testing in Quarkus can sometimes feel overwhelming, with its mix of frameworks and concepts to navigate. In this article, we’ll simplify the process by walking you through the setup of a straightforward integration test for web pages using Playwright, a powerful tool for end-to-end testing.
# Playwright Integration Testing with Quarkus
When developing with Quarkus, you've likely come across the
Getting Started with Testing guide
. If you used the Quarkus CLI to bootstrap your project (quarkus create), it probably generated a simple GreetingResource and an associated integration test.
@QuarkusIntegrationTest
public class GreetingResourceIT extends GreetingResourceTest {
// Run the same tests
}
@QuarkusTest
public class GreetingResourceTest {
@Test
public void testHelloEndpoint() {
given().when()
.get("/hello")
.then()
.statusCode(200)
.body(is("hello"));
}
}
It's a good start but at the same time not ideal is
- The test directly interacts with the actual GreetingResource implementation without mocking dependencies
- It uses RestAssured , which is great for testing APIs but isn’t the best tool for asserting HTML content
- GreetingResourceIT is tightly coupled to GreetingResourceTest, limiting flexibility
# Why Add Playwright?
If your application serves HTML content,
Playwright
is the go-to tool for parsing and asserting DOM elements. It offers robust features for end-to-end testing of web applications. Adding the Playwright dependency can elevate your test coverage and provide a more reliable way to validate HTML output.
<dependency>
<groupId>io.quarkiverse.playwright</groupId>
<artifactId>quarkus-playwright</artifactId>
<version>${quarkus-playwright.version}</version>
<scope>test</scope>
</dependency>
# Using @QuarkusTest
Let’s consider a simple
HomeResource
and its counter part
HomeResourceTest
@Path("/")
@RequiredArgsConstructor
public class HomeResource {
private final IMyService myService;
@GET
@Produces(MediaType.TEXT_HTML)
public TemplateInstance home() {
var content = myService.fetchSomeContent();
return Templates.home().data("content", content);
}
}
@QuarkusTest
@WithPlaywright
public class HomeResourceTest {
@InjectMock
IMyService myService;
@InjectPlaywright
BrowserContext context;
@TestHTTPResource("/")
URL home;
@Test
void home() {
// given
var page = context.newPage();
when(myService.findNextUrl).thenReturn("next-url/go");
// when
var actual = page.navigate(home.toString());
// then
page.waitForLoadState();
assertThat(actual.status()).isEqualTo(200);
assertThat(page.locator(".my-content-link").getAttribute("href")).isEqualTo("next-url/go");
}
}
The resource is exposed at the root level and depends on myService to display some content.
With @QuarkusTest, you can mock out dependencies like myService, allowing you to test the resource in isolation.
Key Points:
- @QuarkusTest is similar to @SpringBootTest in the Spring ecosystem
- Tests run within the same JVM process as the application , enabling bean mocking with @InjectMock
- However, note that these tests don’t run natively—they execute within the JVM
# Using @QuarkusIntegrationTest
Integration tests in Quarkus are different:
- They execute against the native executable and run outside the JVM process
- This approach is ideal for validating your application in production mode.
Challenges:
- Mocking out dependencies becomes more complex in this setup
- You could use profiles ( quarkus.test.integration-test-profile ) to customize behavior, but this typically requires a more elaborate integration environment
@WithPlaywright
@QuarkusIntegrationTest
public class HomeResourceIT {
@InjectPlaywright
BrowserContext context;
@TestHTTPResource("/")
URL home;
@Test
void home() {
// given
var page = context.newPage();
// when
var actual = page.navigate(home.toString());
// then
page.waitForLoadState();
assertThat(actual.status()).isEqualTo(HttpStatus.SC_OK);
assertThat(page.locator(".my-content-link").getAttribute("href")).isEqualTo("next-production-url/go");
}
}
# Conclusion
- Playwright : Consider using it for end-to-end testing, especially for applications serving HTML content
- @QuarkusTest: Easily mock beans by annotating them with @InjectMock for efficient and isolated testing
- @QuarkusIntegrationTest: Best suited for production-like scenarios but has limitations in mocking; profiles can help mitigate this
Leave a thumbs up
Leave a thumbs up if you liked it